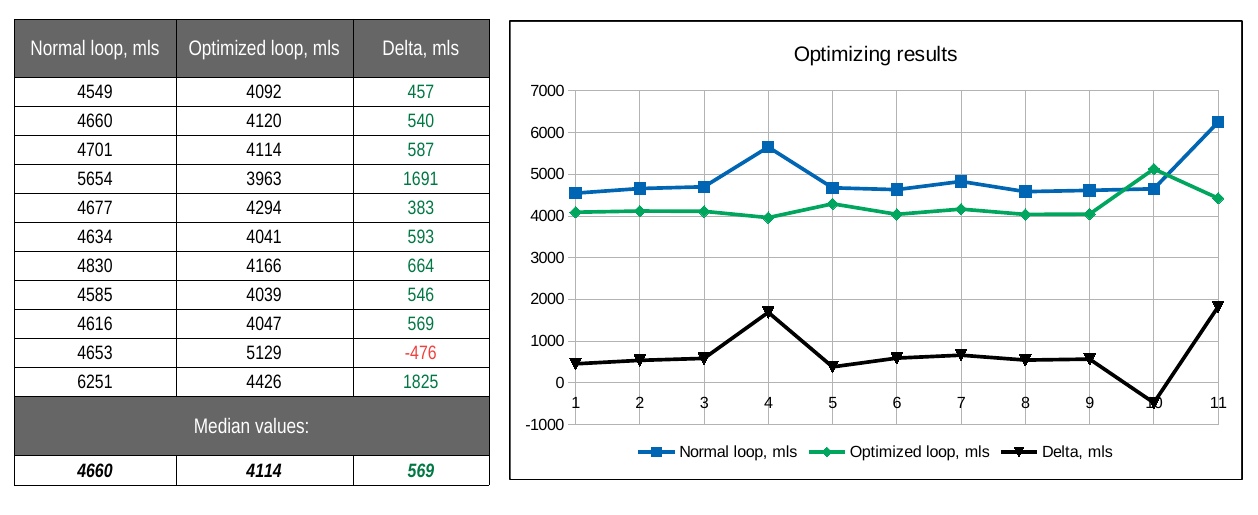
Note
In this article test application listed below was written for J2ME devices and all test results was taken from running application on a real Nokia mobile phone. Applying this optimisation on any other platform or any different language may take effect on the results.
You can try to use optimised loop definitions in PHP, Bash, C++ or any synthax-compatible language, write some tests and check the results.
Basics
Usual loop looks like this:
for (int i = 0; i < 9000000; i++) {
/* do something */
}
So, let’s invert loop counter definition:
for (int i = 8999999; i >= 0; i--) {
/* do something */
}
That’s it. Using this definitions we can write some tests:
import javax.microedition.midlet.*;
public class Application extends MIDlet {
public void startApp() {
long start, end;
/* Обычный цикл */
start = System.currentTimeMillis();
for (int i = 0; i < 9000000; i++) {
/* do something */
}
end = System.currentTimeMillis();
System.out.println("Normal loop: " + (end - start) + " milliseconds");
/* Оптимизированный цикл */
start = System.currentTimeMillis();
for (int i = 8999999; i >= 0; i--) {
/* do something */
}
end = System.currentTimeMillis();
System.out.println("Optimized loop: " + (end - start) + " milliseconds");
System.out.println("\n");
destroyApp(true);
}
public void pauseApp() {
notifyPaused();
}
public void destroyApp(boolean unconditional) {
notifyDestroyed();
}
}